出處: Youtube 彭彭的課程 Java Collection 動態資料結構:雜湊表 HashMap 類別的使用 By 彭彭
實作筆記
最近在上CS 1103 – Programming 的Java課程時覺得感覺到很吃力,很不喜歡uopeople的教課書,用的是這一本Introduction to Programming Using Java,因為uopeople是免學費,所以可能為了要減少學生的支出,所以都使用線上免費的開放教材,但每次選的書我都超不喜歡。所以只好自己上網找喜歡的教材,除了買了洪錦魁老師Java最強入門邁向頂尖高手之路:王者歸來(第二版)全彩版,又在youtube找幾個java的影片,我發現python或是html+css或javascript在Yt都還蠻多教學的,但java相對來說就比較少。
後來在彭彭的課程 發現有兩門Java的hashmap和 Java Thread,都是我不太懂的觀念,而且老師講得不錯,所以就把它寫成筆記紀錄一下啦。
HashMap
HashMap是一個雜湊表。他的儲存內容是key-value,可以從key對應到value一種動態資料結構。
這篇要練習的內容是
- 從檔案讀取字典資料
- 把資料放進HashMap
- 提供字典查詢功能
練習開始
以下是我的資料

執行1.從檔案讀取字典資料
import java.io.*;
import java.util.HashMap;
public class Dictionary {
/* 程式的進入點 */
public static void main(String[] args) throws Exception {
/* 建立HashMap物件 HashMap<KeyDataType,ValueDataType> */
HashMap<String, String> dic = new HashMap<String, String>();
/* 從檔案讀取資料 */
FileReader in = new FileReader("CJ.txt");
BufferedReader reader = new BufferedReader(in); /* 一行一行讀取程式 */
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
/* 提供字典查詢功能,輸入q結束程式 */
}
}
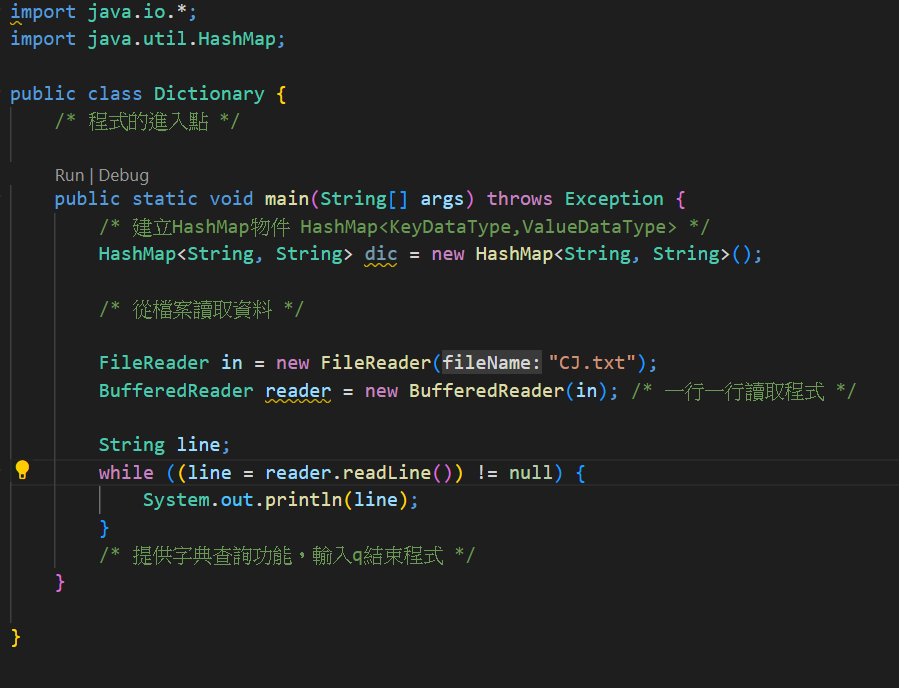
結果
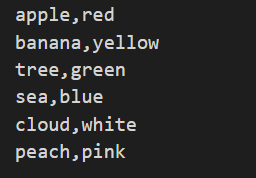
2.把資料放進HashMap
接下執行2.把資料放進HashMap
因為原始資料有一個逗號,所以要把兩筆資料切割開,並放入把資料放進HashMap

import java.io.*;
import java.util.HashMap;
public class Dictionary {
/* 程式的進入點 */
public static void main(String[] args) throws Exception {
/* 建立HashMap物件 HashMap<KeyDataType,ValueDataType> */
HashMap<String, String> dic = new HashMap<String, String>();
/* 從檔案讀取資料 */
FileReader in = new FileReader("CJ.txt");
BufferedReader reader = new BufferedReader(in); /* 一行一行讀取程式 */
String line,fruit,color;
/* 字串的位置所以是int */
int cutIndex;
while ((line = reader.readLine()) != null) {
/* 把逗號裝入cutIndex apple,red */
cutIndex = line.indexOf(",");
/* 把KEY的水果裝入fruit 從開始的第0位到逗號cutIndex */
fruit = line.substring(0, cutIndex);
/* 把Value的顏色裝入color 逗號cutIndex下一位到列的全長 */
color = line.substring(cutIndex+1, line.length());
System.out.println(fruit + "-" + color);
}
}
}
結果
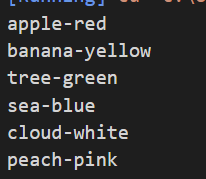
3.提供字典查詢功能
import java.io.*;
import java.util.HashMap;
public class Dictionary {
/* 程式的進入點 */
public static void main(String[] args) throws Exception {
/* 建立HashMap物件 HashMap<KeyDataType,ValueDataType> */
HashMap<String, String> dic = new HashMap<String, String>();
/* 從檔案讀取資料 */
FileReader in = new FileReader("CJ.txt");
BufferedReader reader = new BufferedReader(in); /* 一行一行讀取程式 */
String line,fruit,color;
/* 字串的位置所以是int */
int cutIndex;
while ((line = reader.readLine()) != null) {
/* 把逗號裝入cutIndex apple,red */
cutIndex = line.indexOf(",");
/* 把KEY的水果裝入fruit 從開始的第0位到逗號cutIndex */
fruit = line.substring(0, cutIndex);
/* 把Value的顏色裝入color 逗號cutIndex下一位到列的全長 */
color = line.substring(cutIndex+1, line.length());
/* 把資料放進Hashmap */
dic.put(fruit,color);
dic.put(color,fruit);
}
/* 提供字典查詢功能,輸入q結束程式 */
while(true){
System.out.println("Enter keyword to check:");
String keyword = System.console().readLine();
/* 輸入q跳出迴圈 */
if(keyword.equals("q")){
break;
}
else{
/* 如果key存在,回傳相應的value,否則回傳null*/
String result =dic.get(keyword);
if(result==null){
System.out.println("NO DATA");
}
else{
System.out.println("Your result is "+ result);
}
}
}
}
}

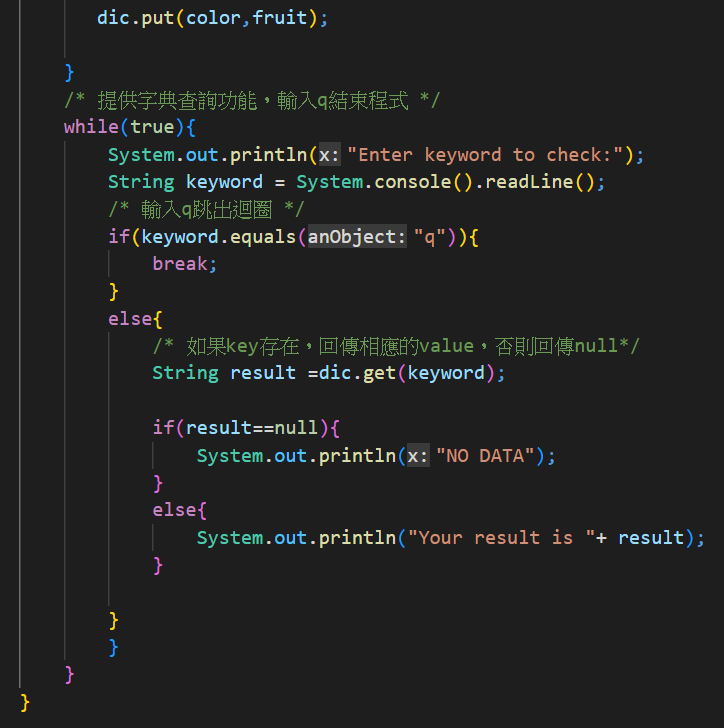
結果
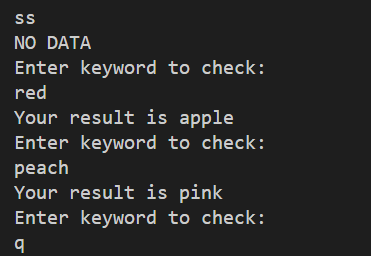
一開始其實運作的沒有很順利,出現了以下錯誤。後來發現我應該在終端機那邊操作而不是輸出那邊。
Exception in thread “main” java.lang.NullPointerException
at Dictionary2.main(Dictionary2.java:31)


執行以下就可以了。

太白癡了,看到大陸網友在CSDN的分享我才找到錯誤,感謝這位網友。
使用Console出现Exception in thread “main“ java.lang.NullPointerException错误