用書 PYTHON王者歸來 作者洪錦魁
函數可以是?
函數可以是一個字串
可用於註解
def Hi(name):
"""input your name"""
print("Say Hello to",name)
Hi("Nini")
print(Hi.__doc__)

執行結果

函數可以是一個物件
大小寫
def upperinput(text):
return text.upper()
print(upperinput("nini"))
a = upperinput
print(a("kini"))
print(upperinput)
print(a)

執行結果

函數可以是資料結構成員
引出內建函數當作串列的元素
def All(a):
return sum(a) *100
x=(1,5,98)
M_list =[min,max,sum,All]
for y in M_list:
print(y,y(x))
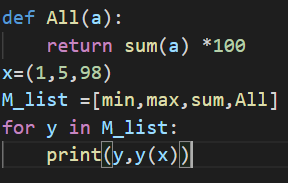
執行結果

函數可以當作參數傳遞給其他函數
def add(a,b):
return a+b
def mul(a,b):
return a*b
def mymath(function1,value1,value2):
return function1(value1,value2)
print(mymath(add,5,9))
print(mymath(mul,5,9))
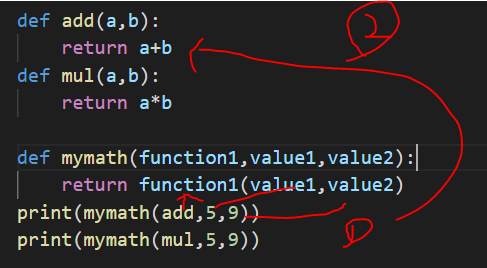
執行結果

函數可以當作參數和不定量的參數*args
def sum1(*args):
return sum(args)
def running(function,*args):
return function(*args)
print(running(sum1,5,6,7))
print(running(sum1,2,3,4,1))
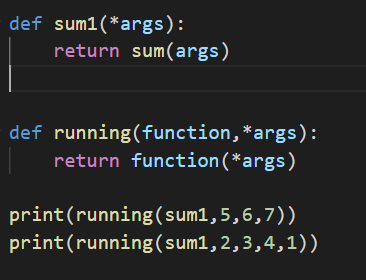
執行結果

勘套函數(函數中的函數)
def mymath1(x1,x2,y1,y2):
def mymath2(z):
return z *5 /2 #*高(5)除2
up = x1-x2 #上底
down= y1-y2 #下底
return mymath2(up + down)
print(mymath1(5,3,9,1))

執行結果

函數可以當作回傳值
內層函數當回傳值的話會傳出內層函數的記憶體位置
def outside():
def inner(a):
return sum(range(a))
return inner
o = outside()
print(o)
print(o(6))
o1 = outside()
print(o1)
print(o1(9)

執行結果

閉包 closure
def outside():
x =15
def inner(b):
return 10*b + x #x =15
return inner
x=2
f = outside()
print(f(x)) #outside(2)
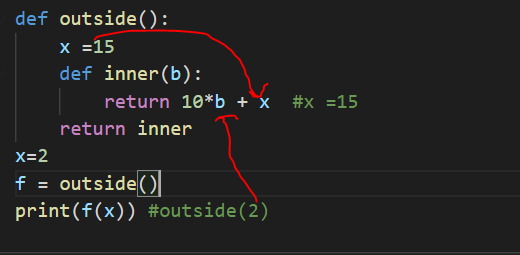
執行結果

def outside(a,b):
def inner(x):
return a*x+b
return inner
f1 = outside(1,9)
f2 = outside(2,8)
print(f1(1),f2(3)) # f1 inner(1) 1*1+9 f2 inner(3) 2*3+8
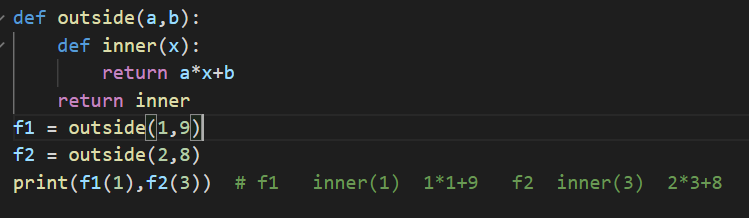
執行結果
