用書 PYTHON王者歸來 作者洪錦魁
裝飾器 Decorate
def lower(func):
def myfuction(args):
old =func(args)
new =old.lower()
print("function name:",func.__name__)
print("function args:",args)
return new
return myfuction
def HI(s):
return s
SayHI = lower(HI)
print(SayHI("How are you NINI?"))
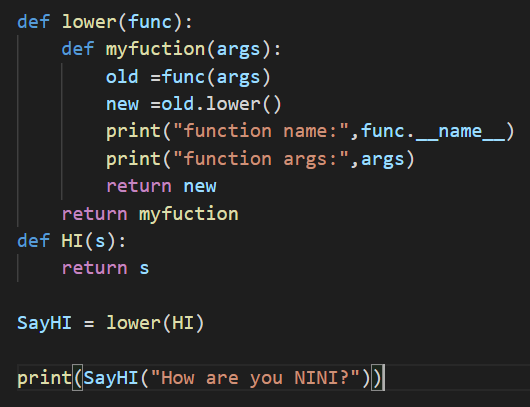
執行結果

def lower(func):
def myfuction(args):
old =func(args)
new =old.lower()
print("function name:",func.__name__)
print("function args:",args)
return new
return myfuction
@lower
def HI(s):
return s
print(HI("How are you NINI?"))

執行結果

def checkerror(func):
def myfunc(*args):
if args[1]!=0:
result =func(*args)
else:
retsult ="除數不為零"
print("function name:",func.__name__)
print("function args:",args)
print("result:",result)
return result
return myfunc
@checkerror
def mymath(a,b):
return a/b
print(mymath(3,2))
print(mymath(3,0))

執行結果
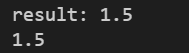

def lower(func):
def myfuction(args):
old =func(args)
new =old.lower()
print("function name:",func.__name__)
print("function args:",args)
return new
return myfuction
def GO(func):
def wrapper(args):
return "------ " + func(args) + "-----"
return wrapper
@GO
@lower
def HI(s):
return s
print(HI("How are you NINI?"))
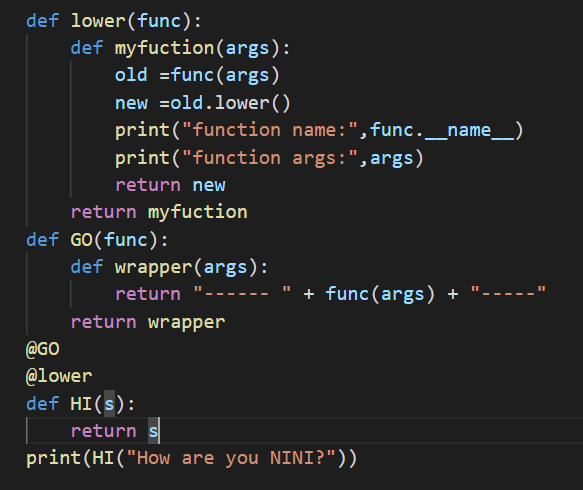
執行結果
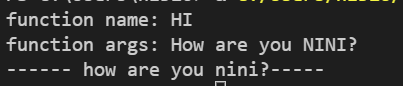
把句點或問號變成空字元+計算出現次數
def modify_classmate_name(names):
for name in names:
if name in ".,?":
names =names.replace(name," ")
return names
def string_to_list(namecount):
global mydict
namelist = namecount.split()
print("name list")
mydict = {wd:namelist.count(wd) for wd in set(namelist)}
classmate_name =""" NINI,Susan Din? Orbi Kan,SISI, susi. Kan Orbi"""
mydict={}
name1 =modify_classmate_name(classmate_name.lower())
print(name1)
string_to_list(name1)
print(mydict)
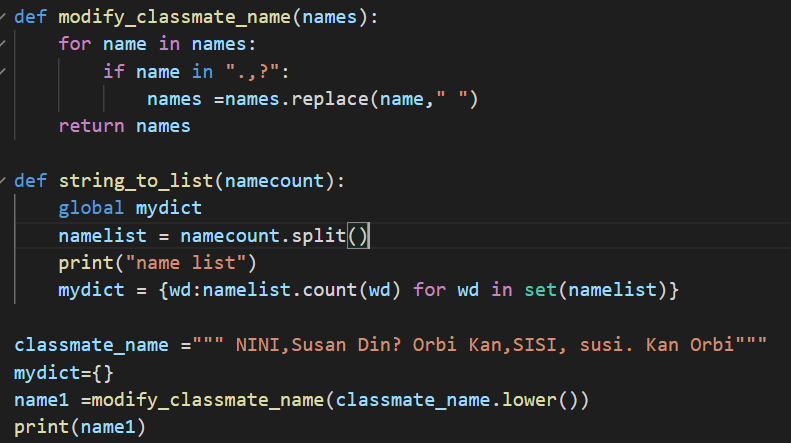
執行結果

回應輸入數字是否為質數
def ismath(num):
for n in range(2,num):
if num % 2 == 0:
return False
return True
num = int(input("Please input a num:"))
if ismath(num):
print("%d is 質數" %num)
else:
print("%d is not 質數" %num)

執行結果
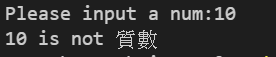

最大公約數
def greatest_common_divisor(n1,n2):
greast = 1
start = 2
while start <= n1 and start <= n2:
if n1 % start ==0 and n2 % start ==0:
greast = start
start += 1
return greast
n1,n2 = eval(input("Please input 2 number:"))
print("greatest_common_divisor is:", greatest_common_divisor(n1,n2))
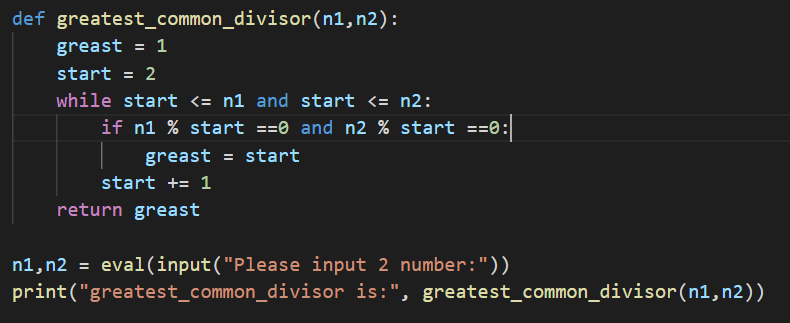
執行結果


輾轉相除法
def greatest_common_divisor(n1,n2):
if n1< n2:
n1,n2 = n2,n1
while n2 !=0:
tmp = n1 % n2
n1 = n2
n2 =tmp
return n1
n1,n2 = eval(input("Please input 2 number:"))
print("greatest_common_divisor is:", greatest_common_divisor(n1,n2))
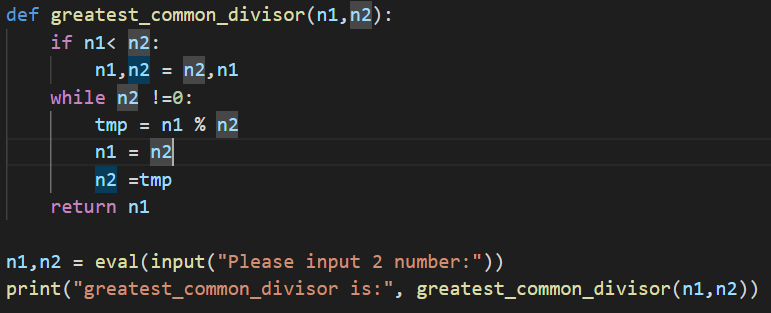
執行結果


遞迴函數處理歐幾里得演算法
def greatest_common_divisor(n1,n2):
return n1 if n2 == 0 else greatest_common_divisor(n2, n1%n2)
n1,n2 = eval(input("Please input 2 number:"))
print("greatest_common_divisor is:", greatest_common_divisor(n1,n2))

執行結果


最小公倍數
def greatest_common_divisor(n1,n2):
return n1 if n2 == 0 else greatest_common_divisor(n2, n1%n2)
def least_common_multiple(n1,n2):
return n1*n2 // greatest_common_divisor(n1,n2)
n1,n2 = eval(input("Please input 2 number:"))
print("greatest_common_divisor is:", greatest_common_divisor(n1,n2))
print("least_common_multiple:", least_common_multiple(n1,n2))

執行結果
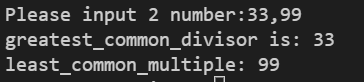
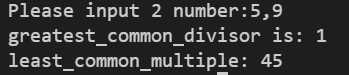